Note
Go to the end to download the full example code
Monte Carlo Quadrature
This tutorial describes how to use Monte Carlo sampling to compute the expectations of the output of a model
using Monte Carlo quadrature applied to an approximation
Monte Carlo quadrature approximates the integral
by drawing
This estimate of the mean,is itself a random quantity, which we call an estimator, because its value depends on the
To demonstrate this phenomenon, we will estimate the mean of a simple algebraic function
where
First setup the example
import numpy as np
import matplotlib.pyplot as plt
from pyapprox.benchmarks import setup_benchmark
np.random.seed(1)
shifts = [.1, .2]
benchmark = setup_benchmark(
"tunable_model_ensemble", theta1=np.pi/2*.95, shifts=shifts)
Now define a function that computes MC estimates of the mean using different sample sets
def plot_estimator_histrogram(nsamples, model_id, ax):
ntrials = 1000
np.random.seed(1)
means = np.empty((ntrials))
model = benchmark.funs[model_id]
for ii in range(ntrials):
samples = benchmark.variable.rvs(nsamples)
values = model(samples)
means[ii] = values.mean()
im = ax.hist(means, bins=ntrials//100, density=True, alpha=0.3,
label=r'$Q_{%d}(\mathcal{Z}_{%d})$' % (model_id, nsamples))[2]
ax.axvline(x=benchmark.fun.get_means()[model_id], alpha=1,
label=r'$Q_{%d}$' % model_id, c=im[0].get_facecolor())
Now lets plot the historgram of the MC estimator
nsamples = int(1e2)
model_id = 0
ax = plt.subplots(1, 1, figsize=(8, 6))[1]
plot_estimator_histrogram(nsamples, model_id, ax)
_ = ax.legend()
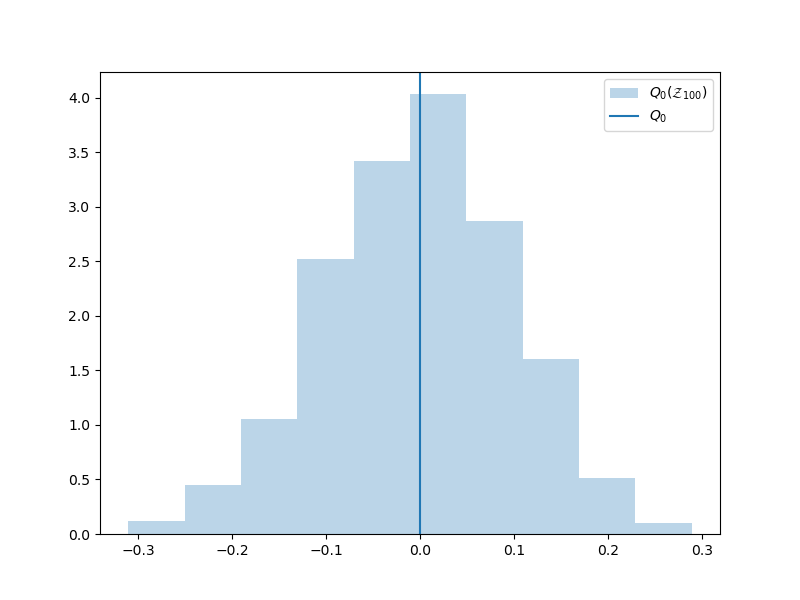
The variability of the MC estimator as we change
model_id = 0
ax = plt.subplots(1, 1, figsize=(8, 6))[1]
nsamples = int(1e2)
plot_estimator_histrogram(nsamples, model_id, ax)
nsamples = int(1e3)
plot_estimator_histrogram(nsamples, model_id, ax)
_ = ax.legend()
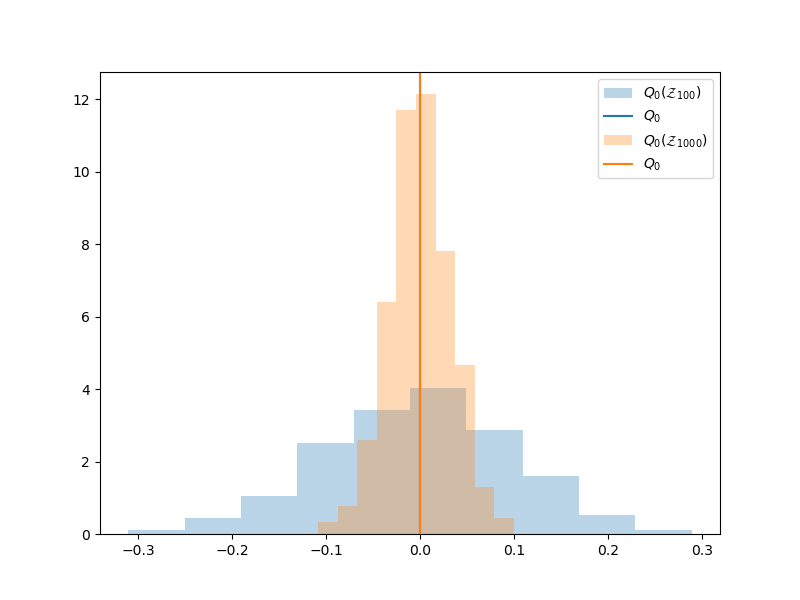
Regardless of the value of
Unfortunately, if the computational cost of evaluating a model is high, then one may not be able to make
model_id = 0
ax = plt.subplots(1, 1, figsize=(8, 6))[1]
nsamples = int(1e2)
plot_estimator_histrogram(nsamples, model_id, ax)
model_id = 1
nsamples = int(1e3)
plot_estimator_histrogram(nsamples, model_id, ax)
_ = ax.legend()
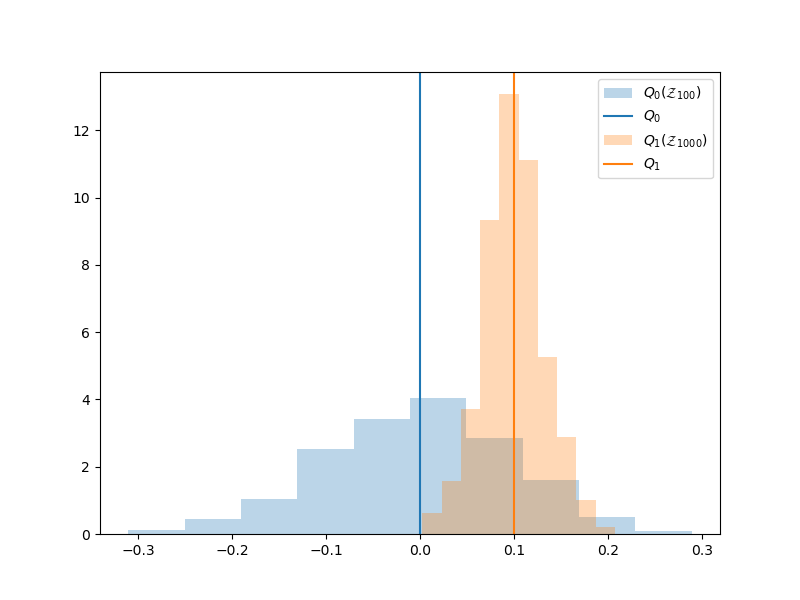
However, using an approximate model means that the MC estimator is no longer unbiased. The mean of the histogram of
Letting
where the expectation
Now using the well known result that for random variable
and the result for a scalar
yields
where
Finally, substituting
From this expression we can see that the MSE can be decomposed into two terms; a so called stochastic error (I) and a deterministic bias (II). The first term is the variance of the Monte Carlo estimator which comes from using a finite number of samples. The second term is due to using an approximation of
Video
Click on the image below to view a video tutorial on Monte Carlo quadrature
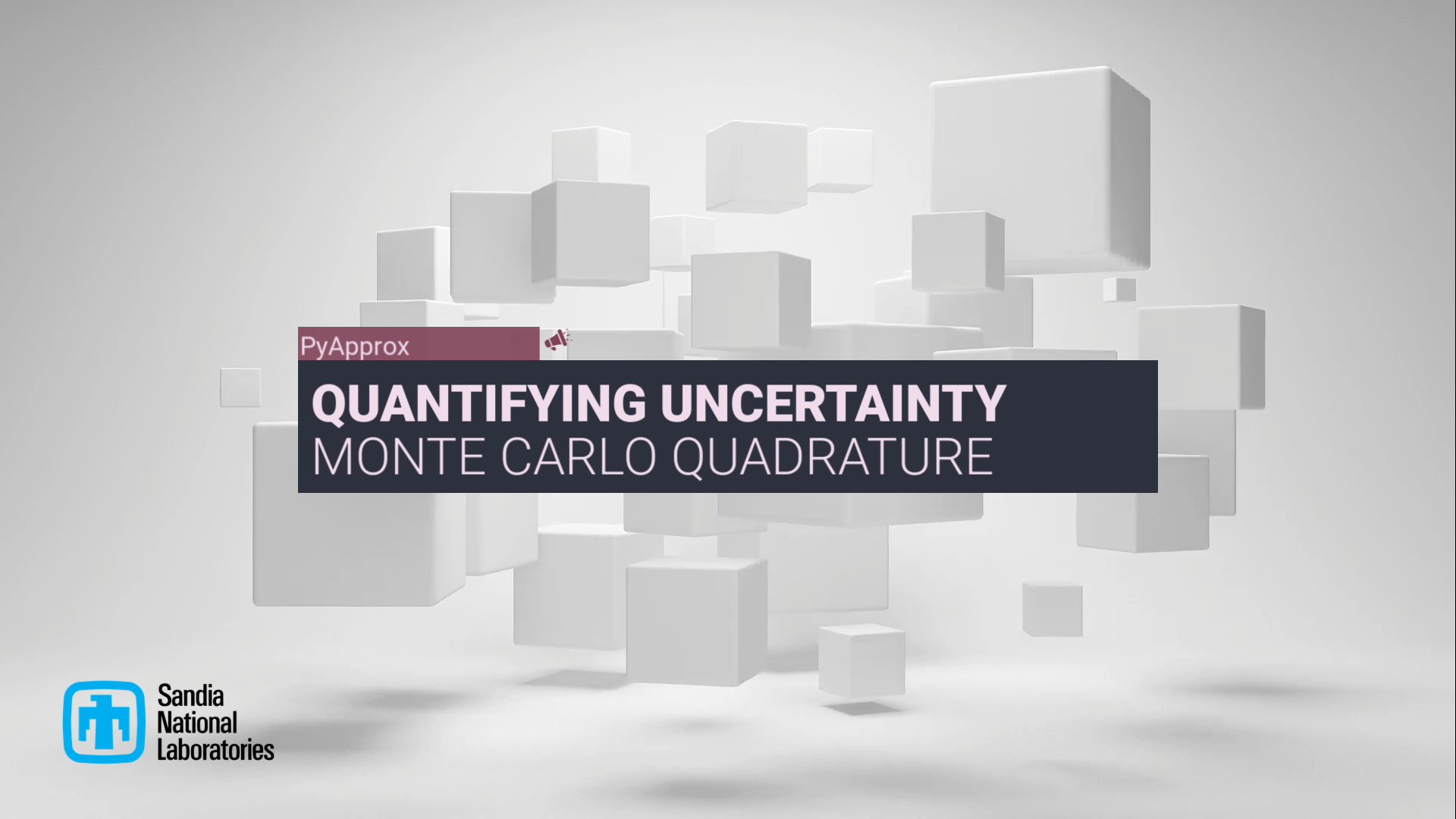
Total running time of the script: ( 0 minutes 0.332 seconds)